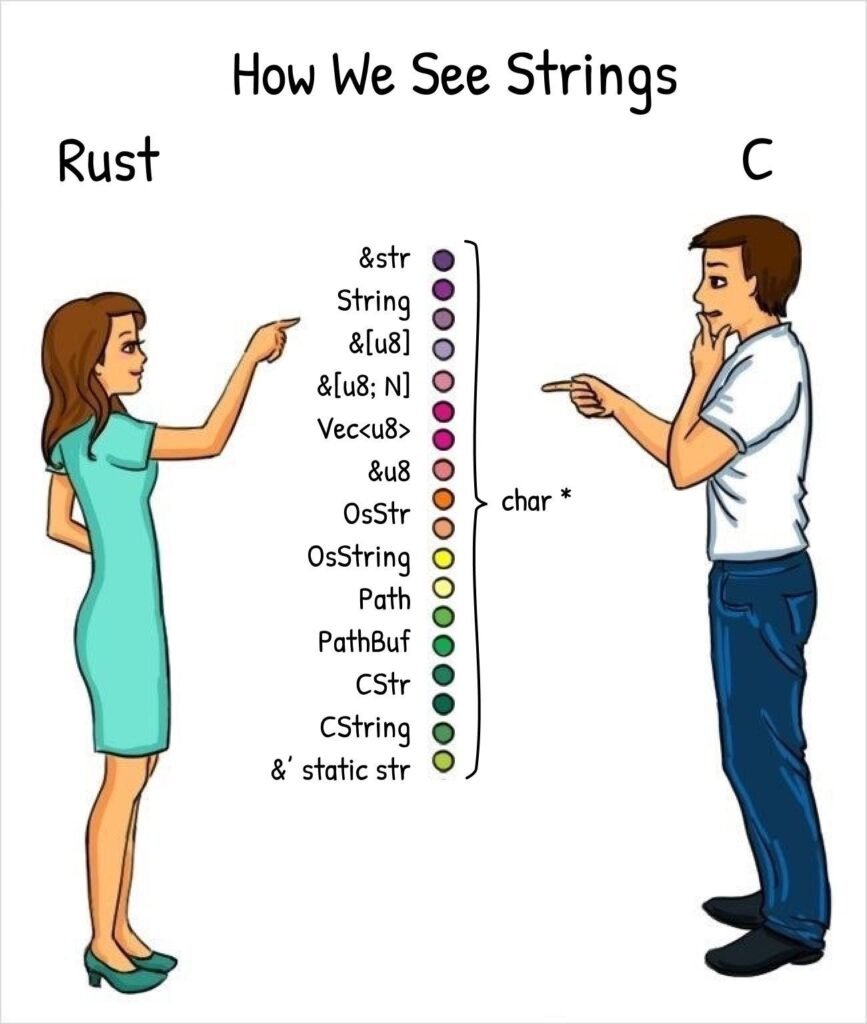
The Colorful Spectrum of Rust Strings
Rust takes a unique approach to strings, offering a wide array of types each suited to different scenarios. This richness stems from Rust’s commitment to safety and efficiency. When you understand the nuances of each type, you’ll be empowered to write Rust code that’s not only correct but also elegantly efficient.
&str
: The Borrowed Slice
We begin with &str
, a borrowed string slice. It’s a view into a string that’s useful for reading data without taking ownership. If Rustaceans had a mantra, it would be to borrow whenever possible. &str
types enforce that at compile-time, ensuring we’re not making unnecessary copies of our strings around the heap.
String
: The Heap Allocated Contender
Next is String
, the growable, modifiable, owned string type. This is the go-to when you need to alter your text on the fly. Under the hood, String
is a wrapper over a Vec<u8>
, making it well-suited for UTF-8 encoded text.
&[u8]
: A Slice of Bytes
When you’re dealing with raw bytes, &[u8]
comes to play. It’s essentially a slice of the Vec<u8>
without any encoding attached. It’s low-level and gives you byte-by-byte control over your data.
Arrays &[u8; N]
: Fixed-Size Byte Buffers
Arrays like &[u8; N]
offer a fixed-size, stack-allocated slice of bytes. They’re blazingly fast but inflexible compared to their growable Vec<u8>
counterparts.
Vec<u8>
: The Flexible Byte Vector
The Vec<u8>
type is a dynamic array of bytes, perfect for when you’re not sure how much data you’re going to have but you know it’s going to be a lot.
OsStr
and OsString
: System-Compatible Strings
The OsStr
and OsString
types come into play when you need to deal with file paths or any other system-based strings. They respect the encoding used by the host OS, which might not be UTF-8.
Path
and PathBuf
: Filesystem Wizards
Path
and PathBuf
are specialized types for dealing with filesystem paths. Path
is to &str
what PathBuf
is to String
: one is borrowed, the other owned. They provide methods tailored for path manipulation, making them indispensable when rummaging through the filesystem.
CString
and CStr
: FFI Friendly Strings
Interoperating with C? CString
and CStr
are your allies, designed to play nicely with C’s null
-terminated strings. They make sure you can cross the FFI boundary without tripping over your strings.
&'static str
: The Immortal Slice
Lastly, &'static str
is a borrowed string slice that’s guaranteed to live for the entire duration of the program. It’s usually hard-coded into the application, and because of its immortality, it can be used anywhere within your Rust code.
The Lone char *
of C
In contrast, C keeps it simple with char *
—a pointer to a character array. It’s the wild west of strings, where you’re responsible for managing memory and ensuring null-termination. It’s the low-level approach that gives you power at the cost of responsibility.
The Rusty Philosophy on Strings
The diversity of string types in Rust isn’t an accident. It’s a carefully crafted design choice that gives you the tools you need for every possible string scenario. From efficient string handling and memory safety to Unicode support and interoperability, Rust’s string types are tailored to fit the precise needs of the task at hand.
When you choose Rust, you’re opting for a language that doesn’t just hope for the best. Instead, it gives you a palette of options, each with its own guarantees and constraints, allowing you to make informed, safe choices.
In summary, Rust’s various string types showcase the language’s dedication to performance, safety, and correctness. It may seem overwhelming at first glance, but once you get the hang of these types, you’ll appreciate the power and flexibility they offer. Whether it’s the efficiency of &str
, the mutability of String
, the OS-level specificity of OsString
, or the interoperability of CString
, there’s a string type for every situation.
Remember, in Rust, strings are not just a sequence of characters; they’re a spectrum of possibilities, each with its place in the Rustacean’s toolbox. Embrace the diversity, and you’ll find yourself writing safer, faster, and more reliable code.
So, to my fellow Rustaceans, next time you’re juggling string types in Rust, appreciate the careful thought that’s gone into giving you just the right tool for your string-slinging needs. And to those still hesitant, take a leap into the Rusty world of strings; it’s colorful, it’s diverse, and it’s meticulously designed for your coding pleasure.