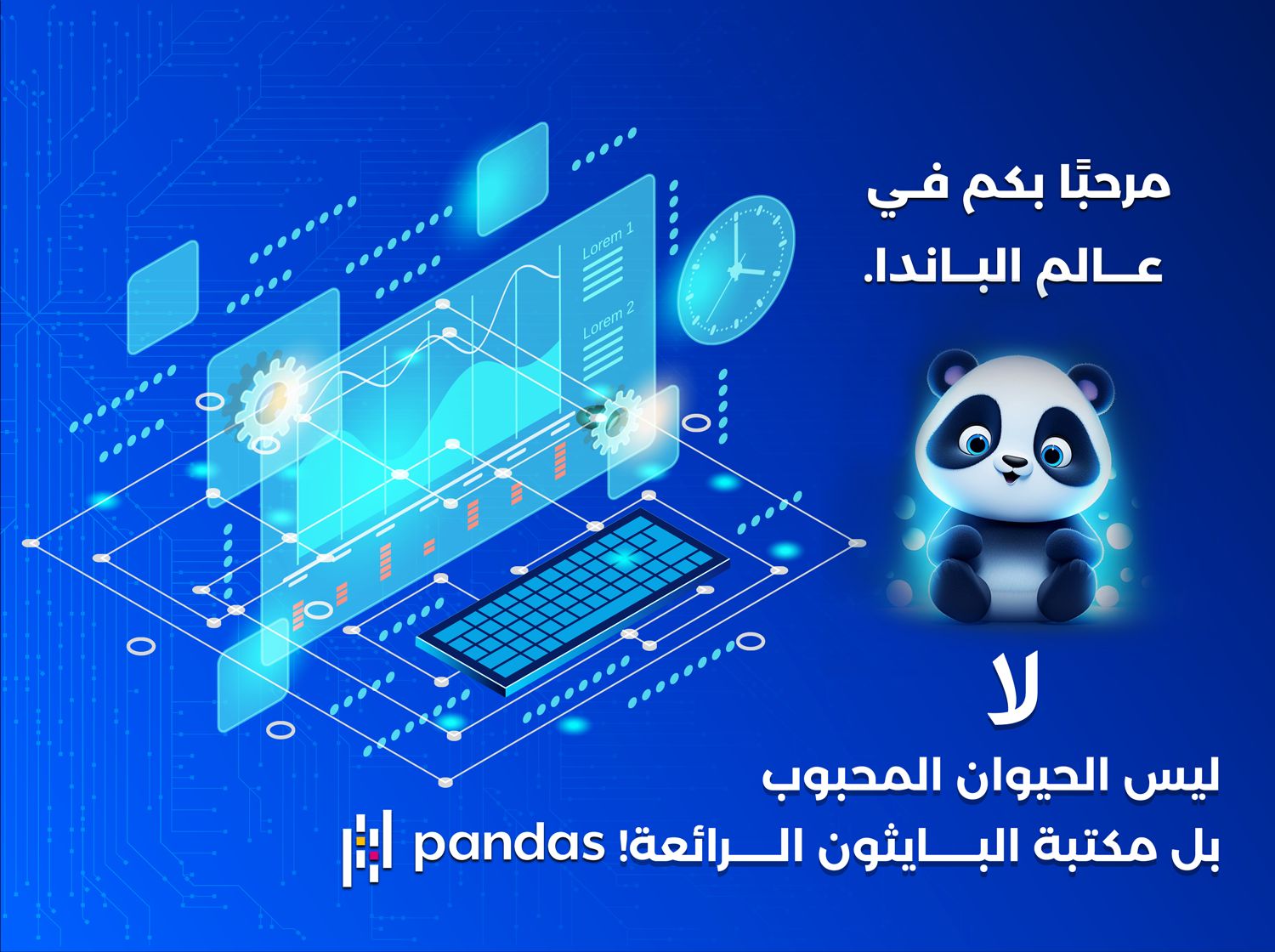
Welcome to the world of Pandas… No, not the adorable animal, but the fantastic Python library! 🐼 If you dream of navigating the data world with ease and elegance, obtaining powerful features and a streamlined code-writing style, then Pandas is your ideal companion on this journey. It’s a programming library considered one of the most important tools in data analysis and handling within the Python programming language, especially in the data field in general.
Why Pandas? 🤔
Simply because it makes complex things… easy!
If you’re wondering how, let’s discover that together in this exciting short journey. Don’t worry, I won’t take much of your time.
Pandas is one of the most important libraries in data science, primarily used for data cleaning, analysis, and exploration. “Panda” is your key to unlocking the doors of the vast and complex world of data, enabling you to handle data in innovative and effective ways while being highly efficient computationally since Pandas relies on the NumPy library for various mathematical operations, which is written in C programming language, offering a significant performance advantage over Python’s default library.
Once you get to know Pandas, you’ll find that working with data is fun and not as complicated as it seems on paper. Are you ready to embark on this journey and discover all that Pandas has to offer? Let’s start this discovery together!
Installing and Importing Pandas 📦
Easy steps to enter the world of Panda
First and foremost, how can we start and enter the world of Pandas? Very easy, all you have to do is install the library and import it into your project. Let’s see how we can do that:
pip install pandas
Don’t worry, the panda won’t bite! Just wait a bit until the installation process is finished, and we’re ready to go.
import pandas as pd
And with this, we’ve taken the first step in the exciting world of Panda!
Pandas Basic Concepts 📚
Let’s talk about data… but in Panda style
DataFrame and Series: In the world of Pandas, there are two main types of data: DataFrame and Series.
- Series: At this stage, it can be considered as a single column of data.
- DataFrame: It’s a data table made up of several columns.
Simply put, we can say that a DataFrame is a collection of columns (Series).
Let’s see how we can create each of them:
import pandas as pd
# Creating a Series
s = pd.Series([1, 2, 3, 4, 5])
# Creating a DataFrame
data = {
'Name': ['Ahmed', 'Mona', 'Omar', 'Sara'],
'Age': [34, 27, 19, 45] }
df = pd.DataFrame(data)
Reading and writing data: You can easily read data from various files like CSV, Excel using Pandas:
# Reading from a CSV file
df = pd.read_csv('path_to_file.csv')
# Reading from an Excel file
df = pd.read_excel('path_to_file.xlsx')
And you can save data in this simple way:
# Saving to a CSV file
df.to_csv('path_to_save.csv', index=False)
# Saving to an Excel file
df.to_excel('path_to_save.xlsx', index=False)
Data Manipulation With Pandas 🔧
Playing around with data using Panda
# Selecting a column
ages = df['Age']
# Adding a column
df['Score'] = [95, 88, 76, 90]
# Deleting a column
df = df.drop(columns=['Score'])
# Filtering data
adults = df[df['Age'] > 18]
# Sorting data
df = df.sort_values(by='Age')
# Describing data
description = df.describe()
Advanced Data Manipulation 🚀
Let’s dive deeper into data analysis with Panda
# Handling missing data
df = df.dropna()
# Filling missing data
df = df.fillna(value=0)
# Merging data sets
merged_df = pd.merge(df1, df2, on='Key_Column')
# Concatenating data sets
concatenated_df = pd.concat([df1, df2])
Let’s make the data speak!
import matplotlib.pyplot as plt
# Plotting data
df['Age'].plot(kind='hist')
plt.title('Age Distribution')
plt.xlabel('Age')
plt.show()
# Box Plot
df.boxplot(column='Age', by='Gender')
plt.title('Age Distribution by Gender')
plt.show()
# Scatter Plot
df.plot(kind='scatter', x='Age', y='Score')
plt.title('Age vs. Score')
plt.xlabel('Age')
plt.ylabel('Score')
plt.show()
After touring the fun world of Panda, are you ready to continue exploring more practical examples and applications? Let’s continue the journey together in the next section!
Real-world Example 🌍
Let’s apply what we’ve learned in a real-life example
Data analysis becomes more fun and useful when we apply it to real-world problems. Let’s take a practical example of how to use Pandas to analyze a real dataset:
import pandas as pd
# Defining the data
data = { 'Name': ['Ahmed', 'Mona', 'Ali', 'Sara', 'Mohamed'],
'Age': [23, 19, 22, 21, 20],
'Score': [89, 92, 78, 85, 95],
'Gender': ['Male', 'Female', 'Male', 'Female', 'Male'] }
df = pd.DataFrame(data)
# Analyzing the data
avg_score = df['Score'].mean()
oldest_student = df.loc[df['Age'].idxmax()]['Name']
highest_scorer = df.loc[df['Score'].idxmax()]['Name']
print(f"The average score is {avg_score:.2f}")
print(f"The oldest student is {oldest_student}")
print(f"The highest scorer is {highest_scorer}")
Conclusion 🎓
What’s next in our journey with Panda?
We’ve come a long way in exploring the world of Pandas, where we learned the basics, moved between data analysis and visual perception, and got a glimpse of how to apply this knowledge to a real problem.
Don’t stop here! The world is full of data and secrets that can be discovered through the use of Pandas. Keep learning and exploring, and you’ll find that Panda will always be there to help you decode the data.
As we’ve learned, Pandas provides the tools you need to analyze data and use it to make informed decisions. Continue to explore more functions and features of Pandas and don’t hesitate to come back and review this post whenever you need!
I hope you enjoyed this journey into the world of Pandas and enjoyed exploring it.
Good job 👏👏🥰